Table of Contents
Embarking on a Java Odyssey: Unveiling the Depths of Java Development
Welcome to a journey through the heart of Java, a language that has been the cornerstone of countless applications, platforms, and systems. Whether you’re a budding coder eager to grasp the basics or a seasoned developer seeking to unravel the intricacies of advanced topics, this blog post is your guide to the expansive world of Java development.
In this odyssey, we will navigate the essentials, explore indispensable tools, uncover best practices, and venture into advanced realms that define the landscape of Java. From the foundational concepts that lay the groundwork to the nuanced intricacies that empower developers, join us as we unravel the layers of Java’s versatility and power. Let’s embark on this Java odyssey together, unlocking the potential of one of the most influential programming languages in the software development cosmos.
Introduction to Java
Java, a programming language that has withstood the test of time, continues to be a cornerstone in the world of software development. Its robust nature, platform independence, and versatility make it a go-to choice for developers across the globe. In this section, we’ll explore the roots, significance, and key features that contribute to Java’s enduring popularity.
The Evolution of Java
Java, developed by James Gosling and Mike Sheridan at Sun Microsystems in the early 1990s, was designed with the vision of creating a language that could run on various devices without recompilation. The language’s journey from its inception to its current state involves consistent updates, improvements, and community contributions. Developers familiar with Java appreciate its commitment to backward compatibility, ensuring that applications developed in older versions continue to function seamlessly.
Importance and Popularity
Java’s prominence in the software development industry can be attributed to its “Write Once, Run Anywhere” (WORA) philosophy. This means that Java code can be written on one device and executed on another without modification. This cross-platform compatibility is invaluable in a world where diversity in devices and operating systems is the norm.
Additionally, Java has been the language of choice for building enterprise-level applications, web services, mobile applications (Android), and more. Its extensive standard library, vast community support, and strong emphasis on security contribute to its widespread adoption.
Key Features of Java
Java’s success lies in its feature-rich architecture that caters to the needs of developers. Some of the key features include:
Object-Oriented Programming (OOP): Java is inherently object-oriented, allowing developers to create modular and reusable code through the use of classes and objects.
Platform Independence: The Java Virtual Machine (JVM) acts as a bridge between the Java code and the underlying hardware, enabling Java applications to run on any device with a compatible JVM.
Automatic Memory Management: Java’s built-in garbage collector automatically manages memory, freeing developers from manual memory management hassles.
Security: Java incorporates robust security features, including a secure runtime environment and built-in APIs for encryption and authentication.
Core Java Concepts
Now that we’ve explored the origins and significance of Java, let’s dive into the foundational concepts that every Java developer must master. These core principles form the backbone of Java programming, providing a solid framework for building robust and scalable applications.
Variables, Data Types, and Operators
At the heart of Java programming are variables, which serve as containers for storing data. Understanding data types is crucial, as they define the kind of values a variable can hold—whether it’s integers, floating-point numbers, characters, or boolean values. The proper use of operators (arithmetic, relational, logical) allows developers to perform various operations on these variables, creating dynamic and interactive programs.
Variables in Java
In Java, a variable is a container for storing data values. Before using a variable, you must declare it with a data type. Here’s a basic example:
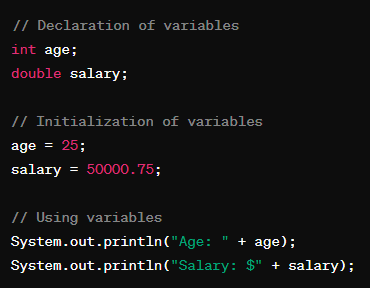
In this example, int
and double
are the data types for the variables age
and salary
, respectively. Java supports various data types such as int
, double
, char
, boolean
, and more.
Data Types in Java
Primitive Data Types
int
: Represents integers (e.g., 5, -10).double
: Represents floating-point numbers (e.g., 3.14, -0.5).char
: Represents a single character (e.g., ‘A’, ‘$’).boolean
: Represents true or false values.
Non-Primitive (Reference) Data Types
String
: Represents a sequence of characters (e.g., “Hello, Java!”).Array
: Represents a collection of elements of the same type.Object
: Instances of user-defined classes.
Operators in Java
Operators are symbols that perform operations on variables and values. Here are some common operators:
Arithmetic Operators
+
(Addition)-
(Subtraction)*
(Multiplication)/
(Division)%
(Modulus)
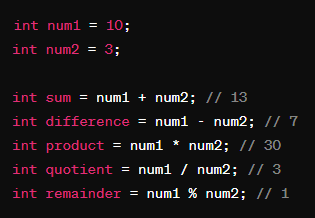
Relational Operators
>
(Greater than)<
(Less than)==
(Equal to)!=
(Not equal to)
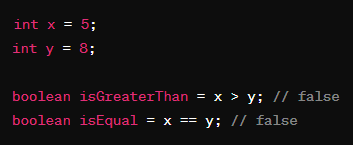
Logical Operators
&&
(Logical AND)||
(Logical OR)!
(Logical NOT)
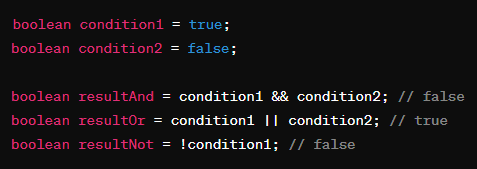
Control Flow Statements
Control flow statements, including if-else conditions, switch cases, and loops, are essential for directing the flow of a program. Conditional statements enable developers to make decisions based on certain conditions, while loops facilitate repetitive tasks. A clear understanding of control flow is fundamental for creating logical and efficient code.
If-Else Statements
Logic: If a certain condition is true, execute a specific block of code; otherwise, execute an alternative block.
Flow
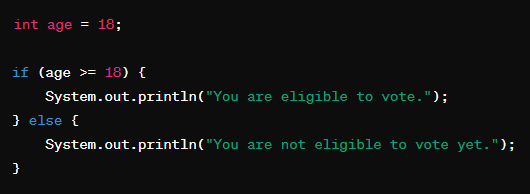
In this example, if the age
is 18 or older, the first block executes; otherwise, the second block executes.
Switch Statement
Logic: Evaluate an expression against multiple possible case values and execute the block of code associated with the first matching case.
Flow
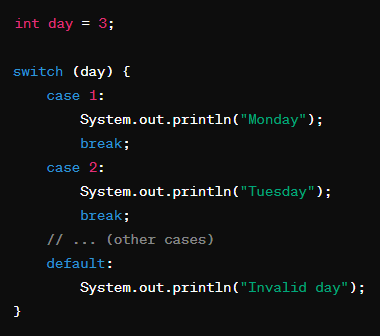
The switch statement checks the value of day
and executes the corresponding block for that case. The break
statement exits the switch.
Loops – For Loop
Logic: Execute a block of code repeatedly until a specified condition is false.
Flow
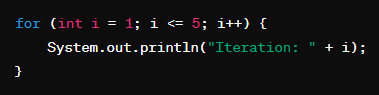
This for loop iterates from i=1
to i=5
, executing the block of code within the loop on each iteration.
Loops – While Loop
Logic: Execute a block of code as long as a specified condition is true.
Flow
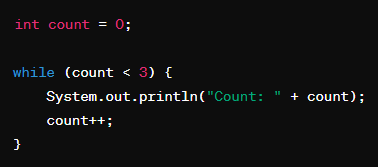
This while loop executes the block of code as long as the count
is less than 3.
Loops – Do-While Loop
Logic: Similar to the while loop, but it always executes the block of code at least once before checking the condition.
Flow

The do-while loop executes the block first and then checks the condition. It continues as long as num
is greater than 0.
Object-Oriented Programming (OOP)
Java’s strength lies in its object-oriented nature. Concepts like classes, objects, inheritance, and polymorphism empower developers to design modular and reusable code. By encapsulating data and behavior within objects, developers can create scalable and maintainable applications.
Classes and Objects
Logic: A class is a blueprint for creating objects. Objects are instances of classes.
Example
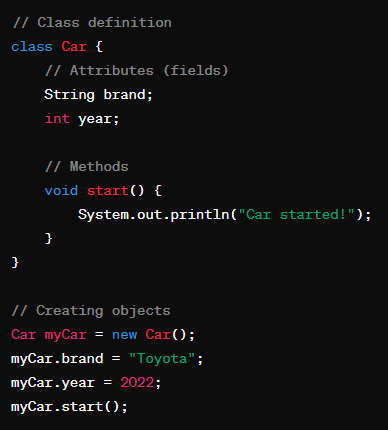
In this example, Car
is a class with attributes (brand and year) and a method (start
). myCar
is an object created from the Car
class.
Encapsulation
Logic: Encapsulation is the bundling of data (attributes) and methods that operate on that data into a single unit (class).
Example
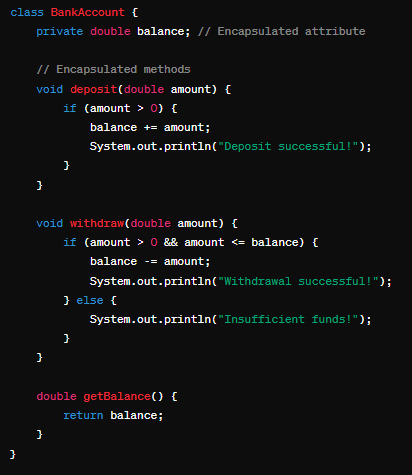
In this example, the balance
attribute is private, and access is controlled through methods (deposit
, withdraw
, and getBalance
), demonstrating encapsulation.
Inheritance
Logic: Inheritance allows a class (subclass) to inherit attributes and behaviors from another class (superclass).
Example
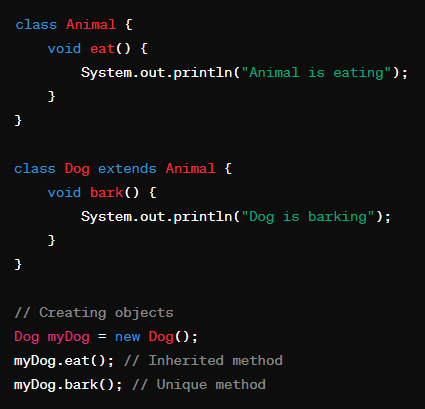
Here, Dog
is a subclass of Animal
. The Dog
class inherits the eat
method from the Animal
class and adds its own method (bark
).
Polymorphism
Logic: Polymorphism allows objects to be treated as instances of their superclass, enabling flexibility in method implementation.
Example
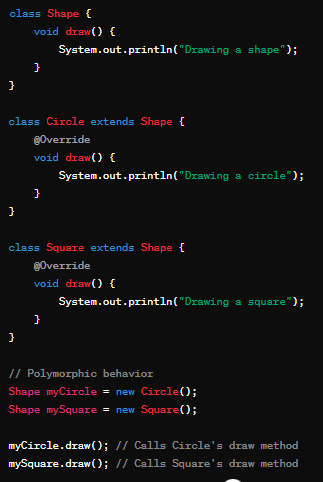
In this example, both Circle
and Square
classes override the draw
method of the Shape
class, and objects of these classes exhibit polymorphic behavior.
Exception Handling and Error Management
In the real world, errors are inevitable. Java provides a robust exception handling mechanism that allows developers to gracefully manage and recover from unexpected situations. Proper error management enhances the reliability of Java applications and ensures a smoother user experience.
Exception Basics
Logic: An exception is an event that occurs during the execution of a program, disrupting the normal flow of instructions.
Example
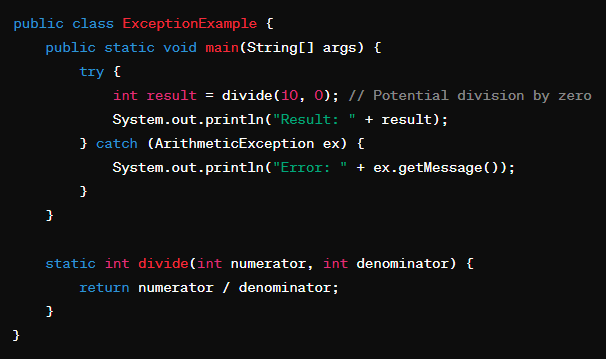
In this example, the divide
method may throw an ArithmeticException
if the denominator is zero. The exception is caught and handled in the catch
block.
Try-Catch Blocks
Logic: The try
block contains code that might throw an exception. The catch
block handles the exception if it occurs.
Example
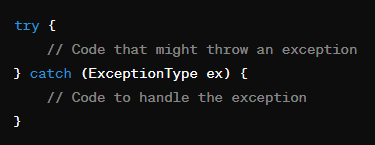
In the previous example, the try
block contains the division operation, and the catch
block handles the ArithmeticException
.
Multiple Catch Blocks
Logic: A try
block can be followed by multiple catch
blocks, each handling a specific type of exception.
Example
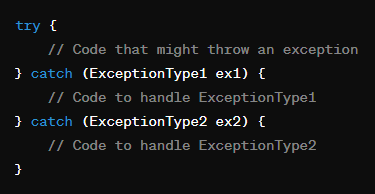
In addition to handling ArithmeticException
, you might have separate catch blocks for other types of exceptions.
Finally Block
Logic: The finally
block contains code that will be executed regardless of whether an exception occurs.
Example
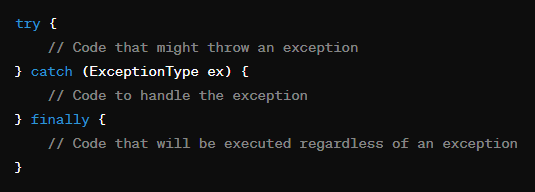
The finally
block is useful for cleanup operations, such as closing resources, that should always be executed.
Throwing Custom Exceptions
Logic: Developers can create and throw custom exceptions to handle specific error scenarios.
Example
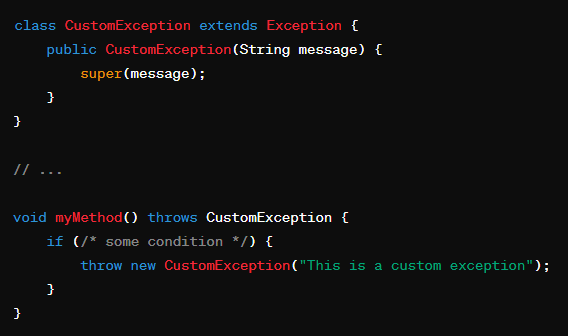
Custom exceptions can be thrown when certain conditions are met, providing more specific information about the error.
Exception Propagation
Logic: If a method does not handle an exception, it is propagated up the call stack until an appropriate catch
block is found.
Example
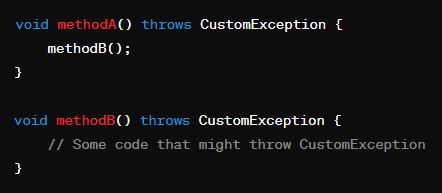
Here, if methodB
throws an exception, it is propagated to methodA
, and so on, until it is caught or reaches the top-level of the program.
Checked vs. Unchecked Exceptions
Logic: Checked exceptions (like IOException
) must be either caught or declared in the method signature. Unchecked exceptions (like RuntimeException
) do not require explicit handling.
Example

Understanding the difference between checked and unchecked exceptions helps in writing effective exception-handling code.
Input/Output Operations (I/O)
Effective communication with external sources is crucial in many applications. Java’s I/O operations enable reading from and writing to various sources, such as files or network connections. Understanding these operations is vital for building applications that interact with the outside world.
Reading Input from the Console
Logic: The Scanner
class is commonly used to read input from the console.
Example
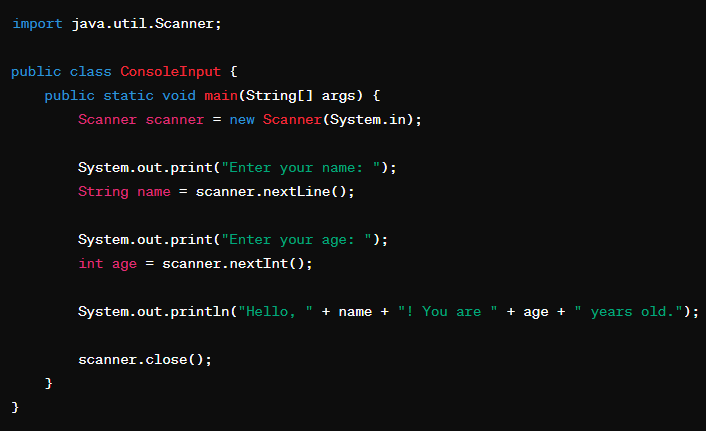
In this example, the Scanner
class is used to read the user’s name and age from the console.
Writing Output to the Console
Logic: The System.out.println
method is commonly used to print output to the console.
Example
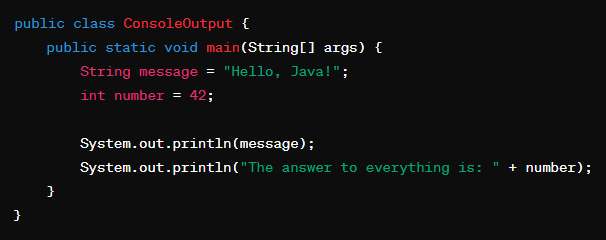
Here, the System.out.println
method is used to display messages and variables on the console.
Reading and Writing Files
Logic: The FileInputStream
and FileOutputStream
classes are used for binary file I/O, while the FileReader
and FileWriter
classes are used for character-based file I/O.
Example

This example demonstrates both writing to and reading from a file using the FileWriter
and FileReader
classes.
Exception Handling in I/O Operations
Logic: I/O operations can throw exceptions (e.g., IOException
). Proper exception handling ensures graceful error management.
Example
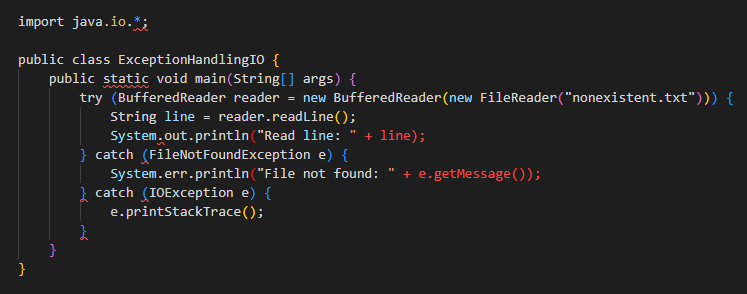
In this example, exception handling is implemented to handle FileNotFoundException
and general IOException
during file I/O operations.
Java Development Tools and Environment
Java development is greatly facilitated by a rich ecosystem of tools and a well-configured development environment. In this section, we’ll explore the key tools and setup that empower Java developers in their coding journey.
Integrated Development Environments (IDEs)
Integrated Development Environments, or IDEs, are comprehensive tools that streamline the development process by providing features like code editing, debugging, and project management.
Eclipse and IntelliJ IDEA
Eclipse and IntelliJ IDEA are two prominent Java IDEs. They offer intelligent code completion, debugging capabilities, and support for various plugins.
Java Development Kit (JDK) and Java Virtual Machine (JVM)
The JDK is a crucial component for Java development, providing compilers, debuggers, and other utilities. The JVM enables cross-platform execution of Java applications.
Setting up JDK
Download and install the JDK from the official Oracle or OpenJDK website.
Step 1: Download JDK
Visit the official Oracle website or the OpenJDK website to download the JDK installer. Choose the appropriate version of JDK based on your operating system (Windows, macOS, or Linux) and system architecture (32-bit or 64-bit).
Step 2: Run the Installer
For Windows
Double-click the downloaded .exe
file.
Follow the on-screen instructions in the installer wizard.
Choose the installation directory and proceed with the installation.
For macOS
Double-click the downloaded .dmg
file.
Drag the JDK package to the Applications folder.
Open a terminal and navigate to /Applications
to access the JDK.
For Linux
Open a terminal.
Navigate to the directory where the downloaded .tar.gz
file is located.
Extract the contents using a command like: tar -zxvf jdk-xx.x.xx_linux-x64_bin.tar.gz
Move the extracted folder to the desired installation location.
Configure the system environment variables like JAVA_HOME
and PATH
for seamless JDK usage.
Step 3: Set Environment Variables (Windows)
For Windows, you need to set the JAVA_HOME
and add the bin
directory to the PATH
environment variable.
Right-click on “This PC” or “Computer” and select “Properties.”
Click on “Advanced system settings” on the left.
Click the “Environment Variables…” button.
Under “System variables,” click “New” to add a new variable.
Variable name: JAVA_HOME
Variable value: Path to the JDK installation directory (e.g., C:\Program Files\Java\jdk-xx.x.xx
).
Find the “Path” variable in the “System variables” section and click “Edit.”
Add a new entry with %JAVA_HOME%\bin
at the end.
Step 4: Verify Installation
Open a new terminal or command prompt and run the following commands:
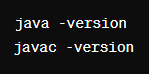
These commands should display the installed Java version and the Java Compiler version, confirming a successful installation.
Version Control Systems
Version Control Systems (VCS) are vital for tracking changes in code, collaborating with team members, and ensuring code integrity.
Git
Git is a widely used distributed version control system. Platforms like GitHub and GitLab host repositories and facilitate collaborative development. Let’s walk through the essential steps to get started with Git.
Step 1: Install Git
For Windows
Download the Git installer from the official Git website:
Run the installer and follow the on-screen instructions. Ensure you select the option to add Git to your system’s PATH during installation.
For macOS
Git is pre-installed on macOS. You can check the version by opening a terminal and typing:
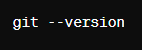
For Linux
Use your package manager to install Git. For example, on Ubuntu or Debian, you can use:
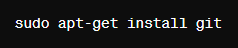
Step 2: Configure Git
After installation, set up your identity by configuring your username and email:

Replace “Your Name” and “your.email@example.com” with your actual name and email.
Step 3: Create a Git Repository
To start version controlling your project, initialize a Git repository in your project’s root directory:

This command initializes a new Git repository in your project.
Step 4: Stage and Commit Changes
Add your files to the staging area to prepare them for commit:
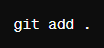
This command stages all changes. Replace the dot (.
) with specific file names to stage only selected files.
Commit the changes to the repository:

The -m
flag allows you to add a commit message directly in the command.
Step 5: Create and Switch Branches
Create a new branch:

Switch to the new branch:

Or, in more recent Git versions:

Step 6: Merge Branches
Merge changes from one branch into another:
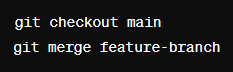
Or, with Git switch:
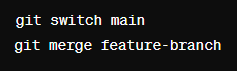
Step 7: Push Changes to Remote Repository
If you’re collaborating with others or using a remote repository (like GitHub), push your changes:
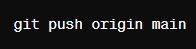
Replace “main” with your branch name.
Step 8: Pull Changes from Remote Repository
To update your local repository with changes from the remote repository:
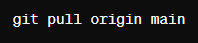
Replace “main” with your branch name.
Build Tools
Build tools automate the process of compiling source code, managing dependencies, and producing executable artifacts.
Maven and Gradle
Maven and Gradle are popular build tools in the Java ecosystem. They simplify project configuration, dependency management, and build processes.
Maven Installation:
Step 1: Download Maven
Visit the official Apache Maven website to download the latest version of Maven:
Step 2: Extract Maven Archive (For Windows)
Once the download is complete, extract the downloaded archive (.zip
file) to a directory on your machine (e.g., C:\Program Files\
).
Step 3: Set Environment Variables (For Windows)
Right-click on “This PC” or “Computer” and select “Properties.”
Click on “Advanced system settings” on the left.
Click the “Environment Variables…” button.
Under “System variables,” click “New” to add a new variable.
Variable name: M2_HOME
Variable value: Path to the Maven installation directory (e.g., C:\Program Files\apache-maven-xx.x.x
).
Find the “Path” variable in the “System variables” section and click “Edit.”
Add a new entry with %M2_HOME%\bin
at the end.
Step 4: Verify Installation
Open a new command prompt and run the following command:

This command should display the installed Maven version, confirming a successful installation.
Gradle Installation:
Step 1: Download Gradle
Visit the official Gradle website to download the latest version of Gradle:
Step 2: Extract Gradle Archive (For Windows)
Once the download is complete, extract the downloaded archive (.zip
file) to a directory on your machine (e.g., C:\Program Files\
).
Step 3: Set Environment Variables (For Windows)
Right-click on “This PC” or “Computer” and select “Properties.”
Click on “Advanced system settings” on the left.
Click the “Environment Variables…” button.
Under “System variables,” click “New” to add a new variable.
Variable name: GRADLE_HOME
Variable value: Path to the Gradle installation directory (e.g., C:\Program Files\gradle-xx.x
).
Find the “Path” variable in the “System variables” section and click “Edit.”
Add a new entry with %GRADLE_HOME%\bin
at the end.
Step 4: Verify Installation
Open a new command prompt and run the following command:
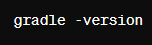
This command should display the installed Gradle version, confirming a successful installation.
Java APIs and Frameworks
In the world of Java development, leveraging Application Programming Interfaces (APIs) and frameworks significantly enhances the efficiency of building robust and scalable applications. Let’s delve into the key concepts surrounding Java APIs and frameworks.
Java APIs
Overview
API (Application Programming Interface): A set of predefined rules, protocols, and tools for building software applications.
Java Standard Edition API (Java SE API): The core API for Java development, offering packages for fundamental functionalities.
Notable Java APIs
Java Collections API: Provides interfaces and classes to represent and manipulate collections (lists, sets, maps, etc.).
Java I/O API: Facilitates input and output operations, including file handling and stream processing.
Java Networking API: Enables communication over networks using protocols like TCP/IP.
Java Frameworks
Overview
Framework: A pre-built, reusable set of abstractions and tools that simplifies complex tasks and enforces a structured development approach.
Java Enterprise Edition (Java EE): A set of specifications, APIs, and frameworks for enterprise-level development.
Popular Java Frameworks
Spring Framework: A comprehensive framework for enterprise J development, addressing various concerns like dependency injection and aspect-oriented programming.
Hibernate: An object-relational mapping (ORM) framework for simplifying database interactions.
Apache Struts: A web application framework for developing Java EE web applications.
Benefits of Using Java APIs and Frameworks
Reusability: APIs and frameworks promote code reuse, reducing development time and effort.
Standardization: Java APIs adhere to standards, ensuring consistency and compatibility across different systems.
Productivity: Frameworks provide ready-made solutions for common tasks, allowing developers to focus on application-specific logic.
Choosing the Right API or Framework
Project Requirements: Assess the specific needs of your project, such as scalability, performance, or integration capabilities.
Community Support: Consider the active community around an API or framework for ongoing support, updates, and troubleshooting.
Learning Curve: Evaluate the learning curve associated with each technology and choose one that aligns with your team’s expertise.
Jav APIs and frameworks play a pivotal role in Java development, offering a structured and efficient approach to building diverse applications. Whether you are working with the core Jav SE API or diving into enterprise-level development with frameworks like Spring, these tools empower developers to create robust and maintainable software solutions.
Best Practices and Advanced Topics
To elevate your Jav development skills and ensure the creation of efficient, maintainable, and scalable code, it’s crucial to adopt best practices and delve into advanced topics. Let’s explore key recommendations and advanced concepts that can enhance your Jav programming proficiency.
Best Practices
Follow Coding Conventions
Best Practice: Adhere to established coding conventions (e.g., Jav Naming Conventions) for consistency and readability.
Why: Consistent coding styles make it easier for developers to understand and collaborate on code.
Effective Use of Collections
Best Practice: Choose the appropriate collection type (List, Set, Map) based on the specific requirements of your data.
Why: Selecting the right collection optimizes performance and ensures efficient data manipulation.
Exception Handling
Best Practice: Handle exceptions gracefully and selectively. Avoid catching generic exceptions unless necessary.
Why: Proper exception handling enhances code robustness and aids in debugging.
Memory Management
Best Practice: Be mindful of memory usage. Dispose of resources properly and consider using tools like the Jav Garbage Collector.
Why: Efficient memory management prevents memory leaks and improves overall application performance.
Advanced Topics
Concurrency and Multithreading:
Advanced Topic: Explore concurrent programming with features like java.util.concurrent
to manage multiple threads.
Why: Utilizing multithreading enhances application responsiveness and performance.
Functional Programming
Advanced Topic: Embrace functional programming concepts with Java 8 features like lambda expressions and the Stream API.
Why: Functional programming enhances code expressiveness and supports concise, elegant solutions.
Design Patterns
Advanced Topic: Learn and apply design patterns (e.g., Singleton, Observer) to solve common design problems.
Why: Design patterns promote code reusability, maintainability, and scalability.
Java Virtual Machine (JVM) Internals
Advanced Topic: Delve into the inner workings of the JVM, understanding concepts like memory areas, garbage collection algorithms, and bytecode execution.
Why: Understanding JVM internals empowers developers to optimize code for performance.
Continuous Learning and Community Engagement
Explore Online Resources
Recommendation: Stay updated with the latest Java features, best practices, and trends by exploring online resources, forums, and blogs.
Why: Continuous learning is essential in a dynamic field like Java development.
Participate in Open Source Projects
Recommendation: Contribute to open source projects to gain real-world experience and collaborate with the broader developer community.
Why: Open source contributions enhance your portfolio and provide valuable exposure to diverse coding styles.
Conclusion
In the vast landscape of Java development, community engagement is a beacon of guidance. Participate in forums, contribute to open-source projects, collaborate with fellow developers and use a proven online courses. The collective knowledge and experience of the Java community serve as a valuable resource for growth.