Table of Contents
Introduction to jQuery
jQuery, a powerful and lightweight JavaScript library, has become an indispensable tool for web developers, streamlining the intricacies of client-side scripting. In this comprehensive guide, we’ll delve into the origins, significance, and key features of jQuery, providing both beginners and seasoned developers with essential insights.
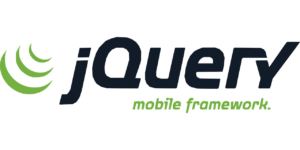
The Birth of jQuery:
jQuery emerged in 2006, conceived by John Resig to address the challenges developers faced in handling JavaScript across various browsers. Its primary goal was to simplify tasks related to DOM manipulation, event handling, and animation, fostering a more efficient and consistent development experience.
Why jQuery Matters in Web Development:
jQuery’s significance lies in its ability to enhance the efficiency of JavaScript coding. It offers a concise and intuitive syntax, allowing developers to achieve complex functionalities with minimal lines of code. By normalizing the intricacies of browser compatibility, jQ facilitates the creation of dynamic and interactive websites across different platforms.
Key Features and Advantages:
DOM Manipulation Made Easy: jQ simplifies DOM manipulation, providing a unified interface for tasks such as selecting, traversing, and modifying HTML elements. This streamlines the process and significantly reduces the code needed for common operations.
Event Handling: Handling user interactions and events is a breeze with jQ. Whether it’s a button click, mouse hover, or keyboard input, jQ simplifies event binding and management, making web development more responsive.
Ajax Support: jQ simplifies the implementation of Ajax (Asynchronous JavaScript and XML) requests, enabling seamless communication with the server. This is crucial for building dynamic and data-driven web applications.
Selecting Elements


Event Handling


DOM Manipulation


Animation


Ajax Request
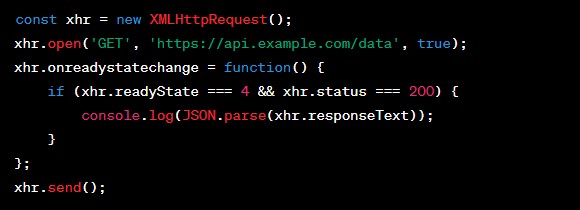

Getting Started with jQuery
jQ serves as a powerful ally for web developers, simplifying the complexities of JavaScript and providing an efficient way to interact with the Document Object Model (DOM). In this segment, we’ll guide you through the initial steps of incorporating jQ into your projects, making the integration process seamless and approachable.
Installation and Setup
Getting started with jQuery is remarkably straightforward. Begin by including the jQ library in your project. You can either download the library from the official website or utilize a Content Delivery Network (CDN) for quicker integration. Here’s a basic example using the CDN:
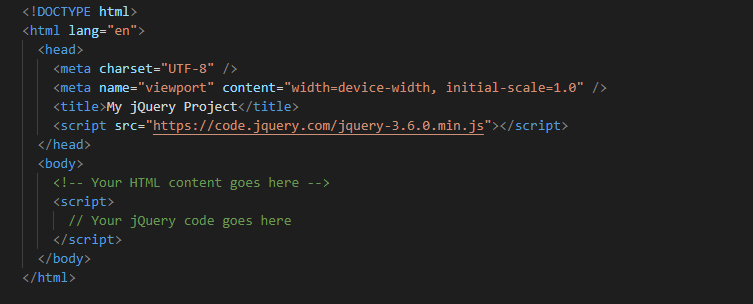
Simply include this script in your HTML file, and you’re ready to start leveraging jQ in your project.
Basic jQuery Syntax
jQ employs a concise and intuitive syntax that simplifies common JavaScript tasks. Let’s take a quick look at a fundamental jQ script:

This snippet ensures that your jQ code executes once the DOM is fully loaded, preventing any potential issues with manipulating elements that haven’t been rendered yet.
DOM Manipulation with jQuery
One of jQuery’s primary strengths lies in its ability to streamline DOM manipulation. Let’s consider a simple example of changing the text content of an element:

In this example, the text content of the element with the ID ‘myElement’ is set to ‘Hello, jQuery!’ using just a single line of code.
Common jQuery Functions
As you delve deeper into jQ, understanding and mastering its common functions is pivotal for efficient web development. In this section, we’ll explore fundamental jQ functions that simplify tasks, providing a concise alternative to traditional JavaScript.
Overview of essential jQuery functions and methods
Certainly! Below is an overview of some essential jQ functions and methods that are commonly used in web development:
Document Ready
The $(document).ready()
function ensures that the code inside it only runs once the DOM (Document Object Model) is fully loaded. It helps prevent issues with manipulating elements that haven’t been rendered yet.

Selectors
jQ selectors allow you to target HTML elements for manipulation. They follow the same syntax as CSS selectors, making them versatile and easy to use
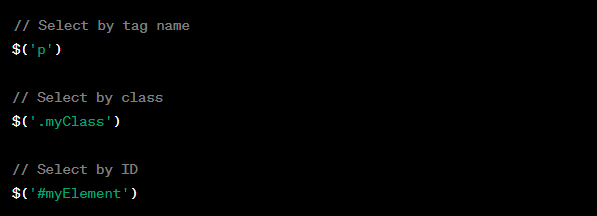
Styling Elements
The css()
method is used to get or set CSS properties of selected elements. It simplifies styling by allowing you to apply changes directly through jQuery.

Changing Text Content
The text()
method is used to get or set the text content of an element. It’s handy for dynamically updating the text of HTML elements.

Modifying HTML Content
The html()
method is similar to text()
, but it allows you to get or set the HTML content of an element. It’s useful when dealing with HTML structure changes.

Manipulating Attributes
The attr()
method is used to get or set attributes of HTML elements. It’s commonly used for handling attributes like src
, alt
, etc.

Fading Elements Out
The fadeOut()
method animates the opacity of selected elements, gradually making them disappear. It’s often used for creating smooth transitions.

Handling Click Events
The click()
method is used to handle the click event on selected elements. It’s a simple way to add interactivity to buttons, links, or any clickable element.

Selecting Elements with jQuery
Selecting HTML elements is a foundational aspect of DOM manipulation. jQ provides versatile selectors that mirror CSS syntax, allowing for precise element targeting. Here’s a quick example:
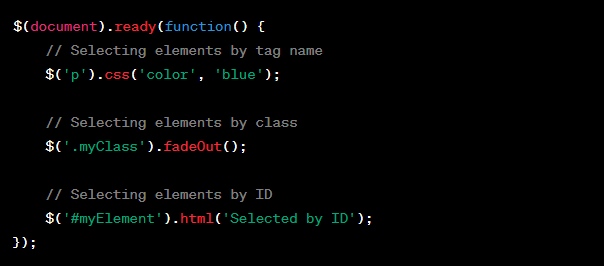
These concise statements demonstrate the flexibility of jQ selectors, making it effortless to apply styles, animations, or content changes to specific elements.
Modifying Content with jQuery
jQ excels in simplifying content modification. Whether you’re altering text, attributes, or HTML structure, jQ provides intuitive methods. Consider the following:
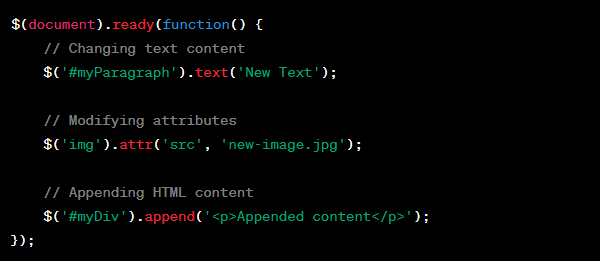
These functions showcase jQuery’s ability to streamline content manipulation, enhancing the developer’s ability to dynamically update and modify the webpage.
Handling Events with jQuery
Event handling is a crucial aspect of interactive web development. jQ simplifies this process with functions like click()
, hover()
, and submit()
. Here’s a basic example:
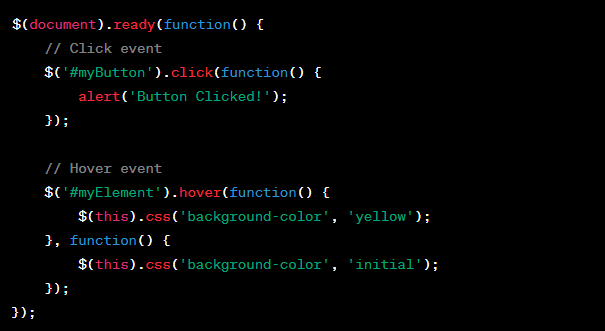
These concise event-handling functions make it easy to define responses to user interactions, adding interactivity to your web pages effortlessly.
Explanation of how jQuery simplifies tasks compared to vanilla JavaScript
jQ simplifies tasks compared to vanilla JavaScript in several ways, making it a popular choice for web developers. Here’s an explanation of some key aspects where jQ offers simplification:
DOM Manipulation
Vanilla JavaScript

jQuery

jQ provides a more concise syntax for selecting and manipulating DOM elements. The chaining of methods allows for multiple operations in a single line, reducing the amount of boilerplate code.
Event Handling
Vanilla JavaScript

jQuery

jQ simplifies event handling by offering shorthand methods like click()
, hover()
, and submit()
. This results in cleaner and more readable code compared to the longer syntax required in vanilla JavaScript.
AJAX Requests
Vanilla JavaScript
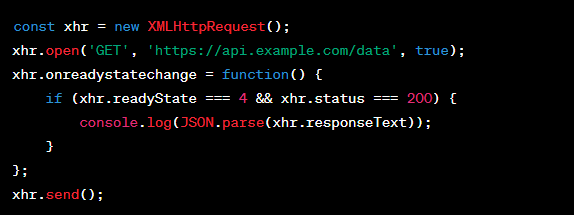
jQuery

jQ simplifies AJAX requests with methods like $.get()
, $.post()
, and $.ajax()
, which handle the complexities of creating XMLHttpRequests. This results in cleaner and more concise code for asynchronous data retrieval.
Cross-Browser Compatibility
Vanilla JavaScript

jQuery

jQ abstracts away browser inconsistencies, providing a consistent API that works seamlessly across different browsers. This simplifies the process of obtaining styles, dimensions, and other properties.
Animation
Vanilla JavaScript

jQuery

jQuery simplifies animation by providing methods like animate()
, which handles the complexities of CSS transitions and animations in a more user-friendly manner.
In summary, jQ simplifies common web development tasks by offering a concise and consistent API, abstracting away cross-browser inconsistencies, and providing shorthand methods for commonly performed operations. While modern vanilla JavaScript has evolved with new features, jQ remains a valuable tool for developers seeking a quick and easy way to achieve common tasks.
jQuery Plugins and Extensions
jQuery’s extensibility is one of its standout features, allowing developers to leverage a plethora of plugins and extensions to enrich their projects. In this section, we’ll explore the world of jQ plugins, highlighting their significance and demonstrating how they can elevate your web development experience.
Understanding jQuery Plugins
jQ plugins are additional scripts that seamlessly integrate with the jQ library, offering ready-made functionalities for common tasks. They provide a modular and efficient way to extend jQuery’s capabilities without the need for extensive custom code.
Integration Process
Incorporating a jQ plugin into your project is typically a straightforward process. Begin by including the plugin script after the main jQuery library in your HTML file. For example:

Once integrated, you can utilize the plugin’s functions within your jQ code, enhancing your project with new features or simplifying complex tasks.
Popular jQuery Plugins
Purpose: Implementing responsive and customizable lightboxes for images, videos, and content.
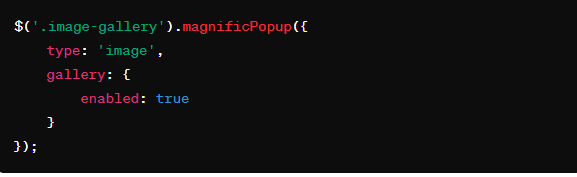
Benefits of jQuery Plugins
Efficiency
jQ plugins eliminate the need to build complex functionalities from scratch, saving development time and effort.
Consistency
Since plugins adhere to the jQ library’s syntax and conventions, they ensure a consistent coding style across projects
Community Support
The vast jQ community contributes to a rich ecosystem of plugins, offering solutions for a wide range of tasks.
Customization
Most plugins come with customizable options, allowing developers to tailor the functionality to suit their specific project requirements.
Best Practices and Tips
jQ, a versatile library, can greatly enhance web development efficiency. To maximize its potential and ensure clean, maintainable code, adopting best practices and following key tips is essential. In this section, we’ll explore some tried-and-true recommendations to optimize your jQ development process.
Keep jQuery Updated
- Best Practice: Regularly update your jQ library to the latest version to benefit from bug fixes, performance improvements, and new features.
- Tip: Utilize a Content Delivery Network (CDN) for efficient library delivery and automatic updates.
Use Efficient Selectors
- Best Practice: Opt for more specific selectors to enhance performance. Avoid overly generic selectors that can impact page rendering speed.
- Tip: Leverage the ID selector for precise targeting when possible, as it is the fastest selector type.
Minimize DOM Manipulations
- Best Practice: Batch DOM manipulations to reduce reflows and repaints. Minimize direct style changes and use CSS classes for bulk updates.
- Tip: Cache frequently used elements to avoid redundant DOM traversals.
Optimize Event Delegation
- Best Practice: Utilize event delegation for efficiency, especially when handling events for multiple elements of the same type.
- Tip: Delegate events to a common ancestor rather than binding them individually to each element.
Use Deferred Objects for Asynchronous Operations
- Best Practice: Embrace jQuery’s Deferred object for better handling of asynchronous tasks like AJAX requests.
- Tip: Leverage promises to improve the readability and maintainability of asynchronous code.
Avoid Global Variables:
- Best Practice: Limit the use of global variables to prevent potential conflicts. Encapsulate your code within closures or utilize the module pattern.
- Tip: Use the
noConflict()
method to relinquish control of the global$
variable if needed.
Optimize Animations
- Best Practice: Use hardware-accelerated animations for smoother performance. Utilize the
requestAnimationFrame
method when applicable. - Tip: Be mindful of excessive animations that may impact user experience.
Avoid Global VEnsure Cross-Browser Compatibilityariables:
- Best Practice: Test your jQ code across multiple browsers to ensure consistent behavior.
- Tip: Leverage feature detection libraries like Modernizr to handle browser-specific functionalities.
Conclusion: Elevate Your Web Development with jQuery Mastery
In the journey through the various facets of jQ, from its fundamental functions to the realm of plugins and best practices, it becomes clear that mastering this powerful library can significantly enhance your web development endeavors. By understanding the simplicity of jQ syntax, exploring its extensive plugin ecosystem, and adopting best practices, developers can unlock a world of efficiency and innovation.
Why Choose jQuery?
jQuery’s enduring popularity is not just a testament to its historical significance but an acknowledgment of its ongoing relevance. While modern JavaScript has evolved, jQ remains an invaluable tool, especially for those aiming to streamline development processes and enhance user experiences.
Moving Forward: Continuous Learning and Innovation
As you embark on your jQ journey or deepen your existing knowledge, remember that the world of web development is ever-evolving. Stay attuned to updates, explore new plugins, and continue adopting best practices to ensure your projects stay at the forefront of innovation.
In closing, jQuery’s versatility, simplicity, and extensive community support make it a formidable ally for developers of all skill levels. Whether you’re building a dynamic website, crafting engaging animations, or optimizing user interactions, jQ provides the tools to elevate your coding prowess.
Embrace the power of jQ, incorporate its best practices, and explore the vast landscape of plugins to create web experiences that captivate and resonate. Your journey into jQuery mastery has just begun, and with each line of code, you’re crafting a more efficient, dynamic, and user-friendly web. Happy coding!